In this project, I made a scene that I named Snowville that is customized based on what the user wants. You can try it out yourself with this link to my code. It is my Christmas themed turtle python project with snow at the top and a person on the very left, some wrapped presents, a snowman, and a Christmas tree with ornaments at the bottom. This was the project that I spent the most time on and it is the one that I am most proud of. Because it is customized, it can be a little different a little time. The main thing I used a lot in my code that I never used before is using the console to have print commands. Then, I have the user type something and based on what they type, the code does a certain thing. I haven’t done that before and that is the main thing I am proud of. It is interactive unlike my other projects. Another thing I used a lot in my code were functions. I did a little bit of functions in my previous projects, but I didn’t understand it that well. While doing this project, I used a lot of functions and that greatly shortened my code. I definitely felt good about that. The code starts out with print commands in the console that greet the user to Snowville. Then, it draws a person over and over again across the screen. I wanted to make it look like a person walking across the screen. After that is the snow. It first draws some snow at the top. Here comes the first customization part. The code asks the user if he/she wants more snow in the scene. If the user types yes, the code will draw more snow, and if he/she says no, then the code will move on. Next is the snowman. The code asks the user what the size of the snowman should be. It gives the options of small, medium, or big. I made it so that it would draw the same snowman but with different sizes based on which size the user types. After that is the presents to the left of the snowman. With this part, the code gives options for what color the presents should be. Like the snowman, I used a function so the code would draw the same objects but the color could vary. The last part of the scene is the Christmas tree. This was the part that took the longest because I decided to have two variables: the color and the size. I had to make multiple functions and different variables. I was eventually able to find a way to include both the color of the ornaments as a variable as well as the size. Here is an example of a possible scene with possible choices:


Let’s look in depth at each of the parts in this code.

First, I imported commands from the turtle and random libraries. Then, I needed the background color to be black so that I could have the things drawn in white be visible. The way to do this is a little weird, but I needed to create a screen variable so I could set the background color. Now, I had to figure out how to use print commands. Just the console printing something is just print, so I started out with that and greeted the user. Then, I needed to find a way to have the user type something and then have to code retain that information to use it later. I looked it up, and I found that I needed to create a variable and do raw_input which would allow the user to type something. Since the thing that the user typed was stored as a variable, I could use it in the line below. I had to print something and the thing that I needed to print was a little complicated. I needed to combine strings of text. This isn’t too hard, but I had to change some of the spacing so it looked like one string of text. After I had a good idea of how to use print commands, it became easier to use them later on. Next, I created the user’s body, which is the white stick figure. I started out making the basic code to draw the figure, but then I wasn’t exactly sure how to make an animation so that it looked like the person was going across the screen. I had a little knowledge of functions, and it seemed that using a function here would make the most sense. That is because, for this animation to work, I would have to draw the person over and over again, just moving him over to the right each time. So making a function would save lots of code. Then, I made a repeat loop because I needed to draw the person and then move him over to the right. That is just what I did. Using a variable for the x position of the body, I was able to move the body over each time it was drawn. Apparently, I looked up that setting the speed to zero means that everything that was supposed to be drawn would be drawn as fast as possible. That was good, because then the drawings of the body would show up faster. So in the end, it just looks like a bunch of bodies on top of each other, but it sort of looks like the body is moving across the screen. It’s sort of an animation. After it drew bodies all the way to the end of the screen, everything was cleared and a body was drawn on the bottom left. That made it look like the person kept walking and now was stepping into a new place. And that place is Snowville.

Now, for the winter/Christmas themed aspects of the project. First, I did the snow at the top. This was pretty simple for me. It was the only time I used random commands since I was randomly putting randomly sized snow at the top. Still, this time the function was a little different. Since I wanted to repeatedly make snow in different places on the screen, I needed parameters in the function since that is the thing that I want to change in the snow function. I decided to randomize the size of the snow since snow doesn’t necessarily drop down in the same size with a variable. Since I made it so that the x and y position of the snow could change, in the repeat loop where I call the function, I made the x and y position randomized in a range so that the snow only appears at the top. Then, I made some basic console exchange between the code and the user. This was the first time I made the code do something based on what the user wanted. Now, this sounded familiar. I asked the user if they wanted more snow or not. If the user said yes, more snow would be drawn, otherwise, it wouldn’t. That would be an if/else command. I used if/else in the past so I knew what to do. I decided to print some text based on the user’s decision to make it more like a conversation and to make it more entertaining.
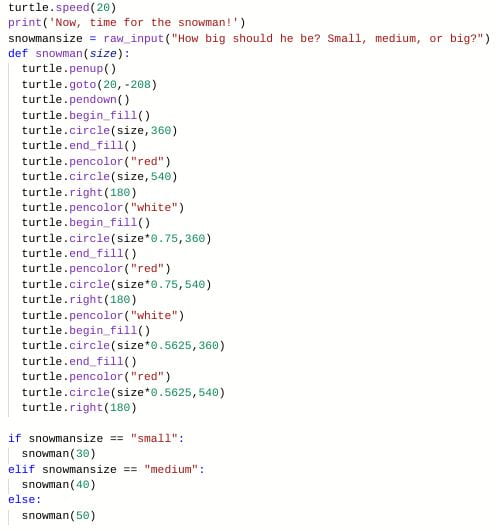
Next, I coded the snowman in the bottom middle. Based on my knowledge when I coded to snow, I already generally knew what to do. Since a snowman is always white, but could be tall or short, the customization I wanted was the size. First, I coded it so it printed three options for the user: small, medium, or big. Based on what the user typed, the same snowman was drawn, just with a different variable. The parameter with this function is the size. The code in the function is a little interesting, since I wanted to use math to have a distinct relationship between the top, middle, and top layers of the snowman and I wanted it to be proportional. So the parameter is the radius of the bottom circle of the snowman, which is the biggest. After it drew the bottom circle, I coded it so the middle circle would be 3/4 the size of the bottom circle. For the top circle, it would by 3/4 the size of the the middle circle, which is 9/16 the size of the bottom circle. This made the snowman look nice. Now, after I ran this, I noticed that the top circle was kind of blending in with the snow. So, to make the snowman stand out more, I created a red outline around the snowman. This made things slightly more complicated. Before, I just had the color set as white and that was it. Now, I needed to switch between white and red. First, the turtle would go around 360 degrees and fill in that circle white. Then, the pencolor would be red and the turtle would go around again, making a red outline. The turtle would end up at the bottom, so I made it 540 degrees to make it travel to the top. It would continue on like that with the middle and top layers. The code in the function is slightly long, but then the if/else part where the code actually runs is super short because I used a function.




After that, I did the presents. This took some thinking, but once it was done, I thought the presents turned out pretty nice. For the presents, the thing I wanted to change was the color, so the user could pick what color their presents should be wrapped in. This one had two functions and then if/else commands to run the code. At first, I wasn’t exactly sure how I wanted to code this and I didn’t really have a clear idea of what I wanted the present portion of the scene to look like. I knew I wanted three presents, but I didn’t know how I would put them together. I just started out getting everything set up so I had the formatting done. I did the print conversation first. Then, I figured, since I was going to be using many different values, it might be easier if I use a list. So the list holds all of the possible colors that the user could chose from. I later named it presenttreecolor because this list is also used for the tree. After that, I created a function for the presents. I made the pencolor white so the outline of the presents would be white and I made a parameter, color, so that I could make the fillcolor the color that the user chooses. I figured out where I wanted the presents to be on the screen and set the x and y position of the the turtle to that using the goto command. Next was just the if/else commands. This was slighly different since I had if the user said a certain color, it would run the presents function with the color corresponding to the list I made. But the if/else part sort of looks more organized because I used the list. Now that everything was formatted, when I ran the code to test out my code for building the presents, it would add the conversation with the user and it would color the presents what the user wanted it to be. Then I started coding the presents because I didn’t want to create a function just yet. I made the first rectangle a horizontal and kind of big sized present. I tried to make sort of an angular present next to that first one, but it didn’t look right to me. So I made a vertical and long rectangle next to the first one. It looked a little weird having presents side by side, so I made a little overlap with the presents so it looked nicer. When I look at these presents, they look a little boring and empty. I thought it would be cool if I could make ribbon sort of things over the presents. This was a little more challenging because I needed to navigate to the middle of a side, change the pen size and pencolor, and go across. I did a little white on the ends of the ribbon because otherwise the ribbons might blend in with the background. At this point I could make a function. For this function, there is three parameters. the length and height are the length and height of the present. The transition parameter is the distance that is the overlap of the presents. The rectangle was straightforward with the parameters. The ribbons, though, were a little tricky. I used the forward commands and the parameters but then I had to modify the parameters, like height/2 to get in the middle of the side. When I got to the ribbon, I needed to change the pensize, go forward a little, change the pencolor to black, go forward the rest of the way minus the little white at the end, change the pencolor back to white, go forward a little, and change the pensize to what it was before. Slightly complicated, but still not too bad. This stage of my project taught me more about making good use of and modifying parameters in functions.

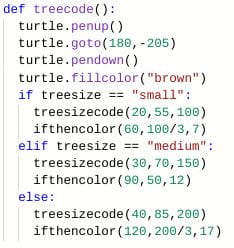




The last thing is the Christmas tree on the bottom right. This part was certainly the longest and hardest part of the code. This is because I wanted the user to get to pick two things: the size of the tree and the color of the ornaments. Figuring out how to combine both of those things was a little tough. First, I made the console code so I could get that done. In the end, I ended up creating four functions just for the tree. Then, all the code runs is just the treecode function! I’m glad that worked out otherwise my code could’ve been really long. I started out just doing the code for the tree without the ornaments. I decided to create the function right away because I felt like I got the hang of the way I used functions in my code. In the function tree code, I got everything set up with the colors and the x and y position. Then, I did the if/else commands for the size and then put the function I was going to put the code for the tree in the if/else commands. For the treesizecode function, there are three parameters. Size1length is the length of the trunk of the tree, size1height is the height of the trunk, and size2 is the length of a side of the green part of the tree (all sides are equal length). So the first part draws the trunk, which is a rectangle. This part is clear. Using the first two parameters, it was easy to make the trunk. Finding out what the values for the parameters were going to be for the different sizes took a bit of trial and error. Then, the transition between the trunk and the green part took some thinking. After the trunk is drawn, the turtle is on the top left corner of the trunk. I needed to get to the point that would be where I would draw the next side. I needed to code the base of the triangle minus the trunk and the part on the right. At first, I thought the formula would be size2/2-size1length, but that would be the wrong order. The bottom side is the length of the trunk, and the remainder of the side. Split that in two and that would be the part I wanted. So the formula is (size2-size1length)/2. Then making the triangle wasn’t hard. It would be size2 and then 120 degree turn, since it is an equilateral triangle, which means it’s also equiangular.
Now, I had to add the ornaments. I wanted to make a different function for the ornaments because I would rather split my code up into more functions that are shorter than have one long function with many parameters. So I made the treecolorcode function for the ornaments. Before I started coding that, I wanted to do the if/else commands which would be for the color. So in each of the if/else commands seeing what the size should be, I needed to put a big if/elif/else command. There are many elif (else if) commands because there are many colors the user could choose from. Now, seeing that there was that if/elif/else command in three different places was a little annoying. I could just make a function for that. So the function, ifthencolor, has that whole part. Then I wouldn’t have to repeat a bunch of code. That was all the functions. The functions are inside one another. Treecolorcode is in the function if thencolor, which is in the function treecode, which is the function that runs. Now, I had to figure out the parameters for treecolorcode.
This is where things started to get more complicated. After the treesizecode function is done, the turtle is on the top right corner of the trunk. I wanted it to be at the bottom left corner of the green triangular part of the tree. This distance is length1. The formula is the whole side of the triangle minus the length of the trunk and minus the whole side minus the length of the trunk divided by 2 (size2-(length1+((size2-length1)/2). Doing some math with the values from the three different sizes, I got the right values and put it in the three corresponding if/else parts in treecode. But then I realized, the function that I put in was ifthencolor, and the function that had the parameters was treecolorcode. So I had to fix that problem. I had to sort of think backwards. Treecolorcode has parameters. The function that runs treecolorcode is ifthencolor. So I needed to set values for the parameters in treecolorcode. But then those values depended on if the size was small, medium, or big. So I found out that I actually had to make parameters that are basically the same as treecolorcode so that I could just put in the values in treecode, where I ran ifthen color. So the values I put in for ifthencolor would be the values for treecolorcode. It was a little confusing at the start because of the functions inside functions thing I was doing, but I got it eventually. Later on, as I was coding treecolorcode, I added two more parameters: length2 and radius. For the ornaments, the turtle would go up 1/3 of the way to the end of the side, make an ornament, and then do that again. So I needed another parameter for that distance, which would just be 1/3 of size2 in my treesizecode function. This distance is length2. The radius is the radius of the circle. Tee size of the circle matters because, for example, if it’s a small tree, there’s got to be smaller ornaments than if it’s a medium sized tree. The first repeat loop is going forward length2 and making circles with a radius of the parameter radius on the left side. Then the second repeat loop is on the right side. I had to make two different repeat loops because the left and right commands are switched. Like I said, quite a bit of thinking and work, but I overcame it.

At the end is just a closing message in the console. So, that is all the work I did for the project. It was very fun to do, I learned a lot more about print commands and interaction with the console as well as applying functions to make code that repeats a lot shorter. Using print commands was always a thing I wanted to do, so I decided to do it in this project. I thought it was an interesting idea. The things that I drew aren’t that detailed or anything, and that is because I just wanted to a little of everything and fill up the screen with those things. Also, I allowed the user to actually sort of have a conversation with the code and have some control over what they wanted to see. Overall, I was very satisfied with my learning experience with this project and with the end result.